This is a reduced version of my Virtual LED Matrix sketch, running on a Wemos D1 Mini @ 80MHz CPU… primarily set to push out a few Blynk commands as fast as possible.
It’s main function timer (LEDTimer) is running some minor internal calculations and then pushes out two Blynk commands and a digital pin toggle ever timed loop. A 2nd timer (CLKtimer) displays the LEDTimer loop and void loop()
counts every second.
In a “normal” setting, I can see this void loop()
issue occurs with the main function timer at around 250ms… giving the timed write loop a cycle of about 5/s and the void loop()
at around 10000-12000/s.
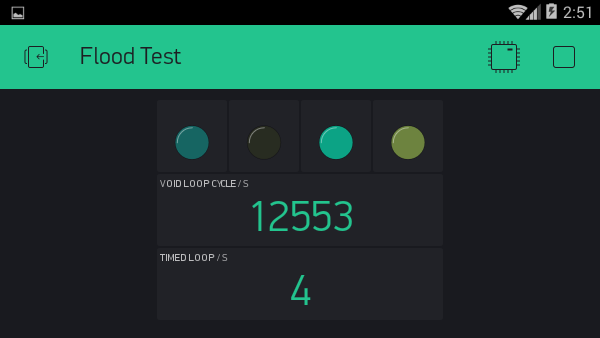
But I have noticed that with a large enough #define BLYNK_MSG_LIMIT 400
, on my Local Server, I can drop the main function timer to around 8-10ms before noting the void loop()
issue, wherein it ranges from around 9000-13000/s to whatever the anti-flooding rate is… usually sub 150/s
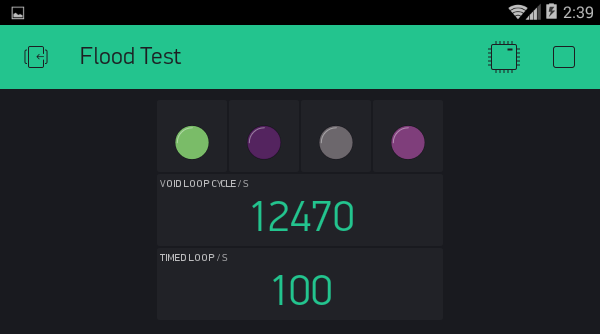
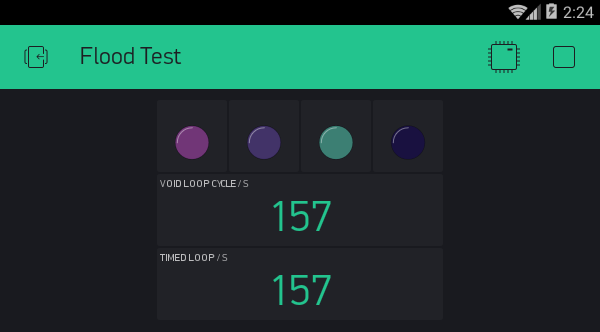
Here is the Flood Test Project files.
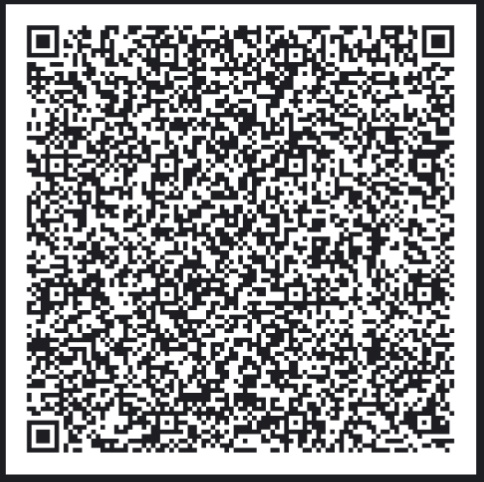
//#define BLYNK_PRINT Serial
//#define BLYNK_MAX_READBYTES 4096
//#define BLYNK_MSG_LIMIT 400 // Uncomment this for faster througput
//#define BLYNK_HEARTBEAT 60
#define BLYNK_NO_BUILTIN // Disable built-in analog & digital pin operations
#include <ESP8266WiFi.h>
#include <ESP8266mDNS.h> // For OTA
#include <WiFiUdp.h> // For OTA
#include <ArduinoOTA.h> // For OTA
#include <BlynkSimpleEsp8266.h>
char auth[] = "xxxxxxxxxx";
char ssid[] = "xxxxxxxxxx";
char pass[] = "xxxxxxxxxx";
String HEXstring;
int randLED, randIntensity, LEDTimer, CLKtimer;
int rrr, ggg, bbb;
int LEDCLK = 0;
int CLK = 0;
BlynkTimer timer;
void setup()
{
pinMode(LED_BUILTIN, OUTPUT);
//Serial.begin(115200);
Blynk.begin(auth, ssid, pass, "xxx.xxx.xxx.xxx", 8442);
LEDTimer = timer.setInterval(250L, RGBMatrix); // Change this for overall speed of LED flashing
CLKtimer = timer.setInterval(1000L, CLKcheck);
ArduinoOTA.setHostname("Flood Test"); // For OTA
ArduinoOTA.begin(); // For OTA
}
void CLKcheck() {
Blynk.virtualWrite(4, CLK);
Blynk.virtualWrite(5, LEDCLK);
CLK = 0;
LEDCLK = 0;
}
void RGBMatrix() {
randLED = random(4);
randIntensity = random(64, 256);
rrr = random(256);
ggg = random(256);
bbb = random(256);
String strRED = String(rrr, HEX); // Convert RED DEC to HEX.
if (rrr < 16) {
strRED = String("0" + strRED); // Buffer with 0 if required.
} // END if
String strGRN = String(ggg, HEX); // Convert GREEN DEC to HEX.
if (ggg < 16) {
strGRN = String("0" + strGRN); // Buffer with 0 if required.
} // END if
String strBLU = String(bbb, HEX); // Convert BLUE DEC to HEX.
if (bbb < 16) {
strBLU = String("0" + strBLU); // Buffer with 0 if required.
} // END if
String HEXstring = String("#" + strRED + strGRN + strBLU); // Combine HEX fragments.
Blynk.setProperty(randLED, "color", HEXstring); // Set random LED HEX colour
Blynk.virtualWrite(randLED, randIntensity); // Set random LED intensity
digitalWrite(LED_BUILTIN, !digitalRead(LED_BUILTIN)); // Flash LED_BUILTIN
LEDCLK++;
}
void loop()
{
CLK++;
Blynk.run();
timer.run();
ArduinoOTA.handle();
}