Here’s some code for you to try (completely untested and written on my phone):
#define BLYNK_PRINT Serial
#include <ESP8266WiFi.h>
#include <BlynkSimpleEsp8266.h>
const int red=D0;
const int green=D1;
const int blue=D2;
int redVal=0;
int greenVal=0;
int blueVal=0;
bool enableRGB = false;
unsigned long time = 0;
int period = 1500;
int offset = 500;
// Your WiFi credentials.
// Set password to "" for open networks.
char ssid[] = "xxxx";
char pass[] = "xxxx";
char auth[] = "xxxx";
BlynkTimer timer;
void stop(){
analogWrite(red, 0);
analogWrite(green, 0);
analogWrite(blue, 0);
}
void rgb(){
if (enableRGB) {
time = millis();
redVal = 128+127*cos(2*PI/period*time);
greenVal = 128+127*cos(2*PI/period*(offset-time));
blueVal = 128+127*cos(2*PI/period*(offset-time));
analogWrite(red, redVal);
analogWrite(green, greenVal);
analogWrite(blue, blueVal);
}
}
BLYNK_WRITE(V5)
{
if(param.asInt() == 1) {
enableRGB = true;
}
else
{
enableRGB = false;
stop();
}
}
void setup()
{
// Debug console
Serial.begin(9600);
pinMode(red, OUTPUT);
pinMode(blue, OUTPUT);
pinMode(green, OUTPUT);
Blynk.begin(auth, ssid, pass);
timer.setInterval(10L, rgb);
}
void loop()
{
Blynk.run();
timer.run();
}
I’ll try your code and tell you if it works well.
but not sure blynk server accept 10L without flooding. 
1 Like
Thanks 
Yeah that may not work nicely with the server and widget LEDs. 
I don’t really like the idea of a timer running every 10ms simply to evaluate an if statement and do the fade routine on the very rare occasions when a fade is actually required.
For a device running 24/7, the fade operation is only going to be needed for a few seconds every day, so asking “are we here yet” every 10ms 24/7 is a bit of an overkill!
A better way would be to create then delete the timer when its needed.
Pete.
2 Likes
you are right @PeteKnight Pete
this is my code with some corrections @dragos
1st you can’t use time, I replace by time1
2nd you have to had a 2nd offset and increase the period
//init
int btnState;
int redVal, greenVal, blueVal;
unsigned long time1 = 0;
int period = 3500;
int offset = 1000;
int offset2 = 2000;
//setup
RGBTimer = timer2.setInterval(10L, rgb);
timer2.disable(RGBTimer);
void rgb() {
if (btnState==true) {
time1 = millis();
redVal = 128 + 127 * cos(2 * PI / period * time1);
greenVal = 128 + 127 * cos(2 * PI / period * (offset - time1));
blueVal = 128 + 127 * cos(2 * PI / period * (offset2 - time1));
red.setValue(redVal);
green.setValue(greenVal);
blue.setValue(blueVal);
}
}
void stop() {
red.setValue(0);
green.setValue(0);
blue.setValue(0);
}
BLYNK_WRITE(V47)
{
if (param.asInt() == 1) {
btnState = true;
timer2.enable(RGBTimer);
}
else
{
btnState = false;
timer2.disable(RGBTimer);
stop();
}
}
1 Like
Wasn’t sure how to enable and disable timers so thanks for showing me too.
Also can you do a gif to show the output of the LEDs as you did before?
as you can see, there is a problem with stop function
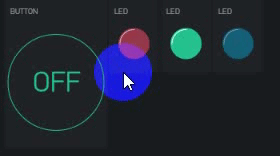
this is my first to compare 
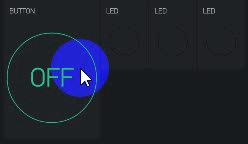
new code to stop led 
BLYNK_WRITE(V47)
{
if (param.asInt() == 1) {
btnState = true;
timer2.enable(RGBTimer);
}
else
{
btnState = false;
timer2.disable(RGBTimer);
timer.setTimeout(2000L, Stop); // <------------- delay to stop the loop
}
}
and now you can play with sliders 
void rgb() {
if (btnState == true) {
time1 = millis();
redVal = 128 + 127 * cos(2 * PI / period * (Speed-time1));
greenVal = 128 + 127 * cos(2 * PI / period * (offset+Speed - time1));
blueVal = 128 + 127 * cos(2 * PI / period * (offset2+Speed - time1));
red.setValue(redVal);
green.setValue(greenVal);
blue.setValue(blueVal);
}
}
BLYNK_WRITE(V28) { //slider
period = param.asInt();
}
BLYNK_WRITE(V29) { //slider
Speed = param.asInt();
}
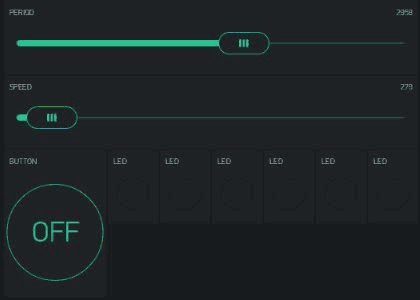
1 Like
I tried most of the codes posted here, the latest try beiing with this one:
const int red=D0;
const int green=D1;
const int blue=D2;
int btnState;
int redVal, greenVal, blueVal;
unsigned long time1 = 0;
int period = 3500;
int offset = 1000;
int offset2 = 2000;
BlynkTimer timer;
//RGBTimer = timer.setInterval(20000L, rgb);
//timer.disable(RGBTimer);
void rgb() {
if (btnState==true) {
time1 = millis();
redVal = 128 + 127 * cos(2 * PI / period * time1);
greenVal = 128 + 127 * cos(2 * PI / period * (offset - time1));
blueVal = 128 + 127 * cos(2 * PI / period * (offset2 - time1));
red.setValue(redVal);
green.setValue(greenVal);
blue.setValue(blueVal);
}
}
void stop() {
red.setValue(0);
green.setValue(0);
blue.setValue(0);
}
BLYNK_WRITE(V2)
{
if (param.asInt() == 1) {
btnState = true;
timer2.enable(RGBTimer);
}
else
{
btnState = false;
timer2.disable(RGBTimer);
stop();
}
}
void setup()
{
// Debug console
Serial.begin(9600);
pinMode(red, OUTPUT);
pinMode(blue, OUTPUT);
pinMode(green, OUTPUT);
Blynk.begin(auth, ssid, pass);
RGBTimer = timer2.setInterval(10L, rgb);
timer2.disable(RGBTimer);
}
void loop()
{
Blynk.run();
timer.run();
}
Still, I am not able to upload it to the board. II’m getting the following error:
request for member ‘setValue’ in ‘red’, which is of non-class type ‘const int’
Am I missing something?
red.setValue(redVal);
green.setValue(greenVal);
blue.setValue(blueVal);
are for ledwidget in my code
WidgetLED red(11);
WidgetLED green(12);
WidgetLED blue(21);
I think you need to replace by something like that
analogWrite(red, redVal);
analogWrite(green, greenVal);
analogWrite(blue, blueVal);
I think you need to replace by something like that
@Blynk_Coeur Yup, you’re right.
‘timer2’ was not declared in this scope
And if I remove these 2 lines:
RGBTimer = timer2.setInterval(10L, rgb);
timer2.disable(RGBTimer);
I get an error for the " timer.run();" inside the loop.
I tried, but I get this instead:
‘RGBTimer’ was not declared in this scope
//Nvm so I declared RGBTimer as int and I have no errors, but it still doesn’t work…
First, use my code with led widget and try to understand it.
Absolutely nothing happens. And the connections are fine, the sliders work well
Can you post exactly what code you have used and maybe a photo of your board/connections?
#define BLYNK_PRINT Serial
#include <ESP8266WiFi.h>
#include <BlynkSimpleEsp8266.h>
char ssid[] = "DMC-2.4G";
char pass[] = "xxx";
char auth[] = "xxx";
const int red=D0;
const int green=D1;
const int blue=D3;
int btnState;
int redVal, greenVal, blueVal;
unsigned long time1 = 0;
int period = 3500;
int offset = 1000;
int offset2 = 2000;
int RGBTimer;
BlynkTimer timer;
BlynkTimer timer2;
void rgb() {
if (btnState==true) {
time1 = millis();
redVal = 128 + 127 * cos(2 * PI / period * time1);
greenVal = 128 + 127 * cos(2 * PI / period * (offset - time1));
blueVal = 128 + 127 * cos(2 * PI / period * (offset2 - time1));
analogWrite(red, redVal);
analogWrite(green, greenVal);
analogWrite(blue, blueVal);
}
}
void stop() {
analogWrite(red, 0);
analogWrite(green, 0);
analogWrite(blue, 0);
}
BLYNK_WRITE(V47)
{
if (param.asInt() == 1) {
btnState = true;
timer2.enable(RGBTimer);
}
else
{
btnState = false;
timer2.disable(RGBTimer);
timer.setTimeout(2000L, stop); // <------------- delay to stop the loop
}
}
void setup()
{
// Debug console
Serial.begin(9600);
pinMode(red, OUTPUT);
pinMode(blue, OUTPUT);
pinMode(green, OUTPUT);
Blynk.begin(auth, ssid, pass);
RGBTimer = timer2.setInterval(10L, rgb);
timer2.disable(RGBTimer);
}
void loop()
{
Blynk.run();
timer.run();
}
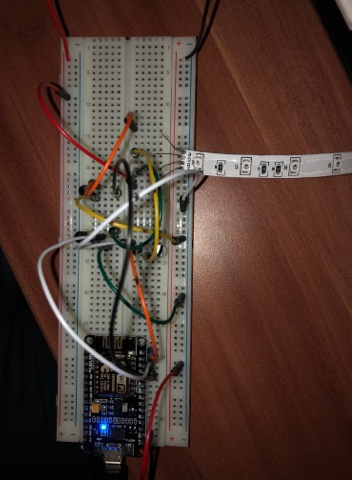
As I said, the connections seem fine as the sliders work and so does the function if I’m using delay instead of simple timer.
//Here’s a more “readable” circuit
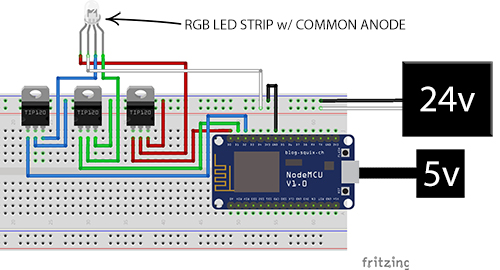
You for forgot
timer2. run
In the loop
Else replace all "timer2 " by “timer”